변수의 데이터 타입은 7가지로 되어있다.
- 숫자(Number)
- 문자열(String)
- 불리언(Boolean)
- undefined
- null
- 객체(Object)
- 배열(Array)
하나씩 알아보도록 하자
1. 숫자(Number)
변수를 선언하고, 값을 할당할 때 따옴표(' ' / " ") 로 감싸서 표현하면 데이터 타입이 문자열로 된다.
따라서, 숫자값을 할당하고자 한다면 따옴표 없이 할당하도록 하자.
1-1. 정수형 숫자 (Integer)
let num1 = 10;
console.log(num1); // 10
console.log(typeof num1); // "number"
num1 변수에 따옴표 없이 10을 할당 후 로그를 찍어보면 10이 출력되며
데이터 타입은 숫자(number) 가 된다.
let num2 = "10";
console.log(num2); // 10
console.log(typeof num2); // "string"
num2 변수에 따옴표와 함께 10을 할당 후 로그를 찍어보면 10이 출력되며
데이터 타입은 문자열(string) 이 된다.
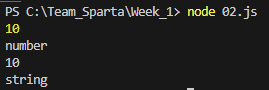
1-2. 실수형 숫자 (Float)
let num1 = 3.14;
console.log(num1); // 3.14
console.log(typeof num1); // "number"
num1 변수에 따옴표 없이 3.14를 할당 후 로그를 찍어보면 3.14가 출력되며
데이터 타입은 숫자(number) 가 된다.
let num2 = "3.14";
console.log(num2); // 3.14
console.log(typeof num2); // "string"
num2 변수에 따옴표와 함께 3.14를 할당 후 로그를 찍어보면 3.14가 출력되며
데이터 타입은 문자열(string) 이 된다.
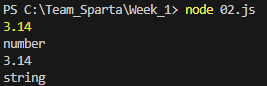
1-3. 지수형 숫자 (Exponential)
let num1 = 2.5e5; // 2.5 x 10^5
console.log(num1); // 250000
console.log(typeof num1); // "number"
num1 변수에 따옴표 없이 2.5e5를 할당 후 로그를 찍어보면 250000가 출력되며
데이터 타입은 숫자(number) 가 된다.
let num2 = "2.5e5"; // 2.5 x 10^5
console.log(num2); // 2.5e5
console.log(typeof num2); // "string"
num2 변수에 따옴표와 함께 2.5e5를 할당 후 로그를 찍어보면 2.5e5가 출력되며
데이터 타입은 문자열(string) 가 된다.
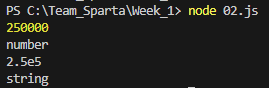
1-4. NaN (Not a Number, 숫자가 아님)
let num1 = "Hello" / 2;
console.log(num1); // NaN
console.log(typeof num4); // "number"
num1 변수에 따옴표와 함께 "Hello" / 2를 할당 후 로그를 찍어보면 NaN이 출력되며
데이터 타입은 숫자(number) 가 된다.
Hello 문자열을 2로 나눈다는 말도 안되는 식을 작성하여 숫자가 아님을 뜻하는 NaN이 출력되었다.
NaN(Not a Number)은 자바스크립트에서 숫자가 아님을 나타내는 값입니다.
보통 수학적으로 정의되지 않는 계산을 수행하거나, 숫자가 아닌 값을 숫자로 변환하려고 할 때 발생합니다.

1-5. 무한대 (Infinity)
let num1 = 1 / 0;
console.log(num1); // Infinity
console.log(typeof num1); // "number"
num1 변수에 1을 0으로 나누는 식을 할당 후 로그를 찍어보면 Infinity가 출력되며
데이터 타입은 숫자(number) 가 된다.
let num2 = -1 / 0;
console.log(num2); // -Infinity
console.log(typeof num2); // "number"
num2 변수에 -1을 0으로 나누는 식을 할당 후 로그를 찍어보면 -Infinity가 출력되며
데이터 타입은 숫자(number) 가 된다.
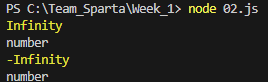
2. 문자열(String)
let str = "Hello, world!";
console.log(str); // Hello, world!
str 변수에 "Hello, world!" 를 할당 후 로그를 찍어보면 Hello, world!가 출력되며
데이터 타입은 문자열(string) 가 된다.
변수를 선언하고, 값을 할당할 때 따옴표(' ' / " ") 로 감싸서 표현하면 데이터 타입이 문자열로 된다.
따라서, 문자열값을 할당하고자 한다면 따옴표 없이 할당하도록 하자.
2-1. 문자열 길이(Length) 확인하기
let str = "Hello, world!";
console.log(str.length); // 13
str 변수에 "Hello, world!" 를 할당 후 로그를 찍어보면 13이라는 숫자가 출력된다.
변수 str이 가지고 있는 문자열의 길이가 13이라는 뜻이다.
lenth를 사용하면 변수가 가지고 있는 길이를 확인할 수 있다.

2-2. 문자열 결합(Concatenation)
let str1 = "Hello, ";
let str2 = "world!";
let result = str1.concat(str2);
console.log(result); // "Hello, world!"
str1 변수에 "Hello, " 를 할당, str2 변수에 "world!" 를 할당
result 변수에 str1.concat(str2)를 할당 후 로그를 찍어보면 Hello, world!가 출력된다.
concat을 사용하면 문자열을 결합하여 준다.

2-3. 문자열 자르기(Substring, Slice)
let str = "Hello, world!";
console.log(str.substr(7, 5)); // "world"
console.log(str.slice(7, 12)); // "world"
str 변수에 "Hello, world!" 를 할당 후 로그를 찍어보면 동일하게 World가 출력된다.
substr 은 시작위치부터, 몇개까지 자르는 기능이다.
slice 는 시작 위치부터 끝 위치 까지 자르는 기능이다.

2-4. 문자열 검색(search)
let str = "Hello, world!";
console.log(str.search("world")); // 7
str 변수에 "Hello, world!" 를 할당 후 로그를 찍어보면7 이라는 숫자가 출력된다.
search 는 지정한 문자열이 시작되는 지점을 찾아주는 기능이다.

2-5. 문자열 대체(replace)
let str = "Hello, world!";
let result = str.replace("world", "JavaScript");
console.log(result); // "Hello, JavaScript!"
str 변수에 "Hello, world!" 를 할당
result 변수에 "world", "JavaScript" 를 할당 후로그를 찍어보면
Hello, JavaScript! 가 출력된다.
replace 는 지정한 문자열을 변경하는 기능이다.

2-6. 문자열 분할(split)
let str = "apple, banana, kiwi";
let result = str.split(",");
console.log(result); // ["apple", " banana", " kiwi"]
str 변수에 "apple, banana, kiwi" 를 할당
result 변수에 str.split(",") 를 할당 후 로그를 찍어보면
배열 형태인 ["apple", " banana", " kiwi"] 가 출력된다.
split 은 지정한 스플리터(splitter)를 기준으로 문자열을 나누고 배열에 저장한다.

3. 불리언(Boolean)
let bool1 = true;
console.log(bool1); // true
console.log(typeof bool1); // "boolean"
bool1 변수에 true를 할당 후 로그를 찍어보면 true가 출력되며
데이터 타입은 불리언(Boolean) 이 된다.
let bool2 = false;
console.log(bool2); // false
console.log(typeof bool2); // "boolean"
bool2 변수에 false를 할당 후 로그를 찍어보면 false가 출력되며
데이터 타입은 불리언(Boolean) 이 된다.
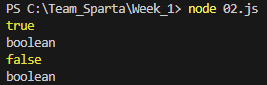
불리언은 참(true)과 거짓(false)을 나타내는 데이터 타입이다.
불리언 데이터 타입은 조건문(if, else, switch 등)과 논리 연산자(&&, ||, !)와 함께 많이 사용된다.
예를 들어, 아래와 같은 코드를 작성할 수 있다.
let x = 10;
let y = 5;
if (x > y) {
console.log("x is greater than y");
} else {
console.log("x is less than or equal to y");
}
let a = true;
let b = false;
console.log(a && b); // false
console.log(a || b); // true
console.log(!a); // false
위 코드에서는 if 조건문을 사용하여 x가 y보다 큰 경우에는 "x is greater than y"를 출력하고, 그렇지 않은 경우에는 "x is less than or equal to y"를 출력합니다. 또한, 논리 연산자를 사용하여 a와 b의 논리적인 AND(&&)와 OR(||) 연산을 수행하고, NOT(!) 연산을 수행합니다.
4. undefined
let x;
console.log(x); // undefined
console.log(typeof x); // undefined
x 변수에 아무것도 할당하지 않은 후 로그를 찍어보면 undefined가 출력되며
데이터 타입은 언디파인(undefined) 이 된다.

undefined는 값이 할당되지 않은 변수를 의미한다.
코드를 작성 하다가 undefined가 나왔다면, 변수에 값이 할당되지 않았다는 뜻으로, 작성한 코드를 살펴보자.
5. null
let x = null;
console.log(x); // null
console.log(typeof x); // object

null은 값이 존재하지 않음을 의미하며, 값이 존재하지 않음을 명시적으로 나타내는 방법이다.
명시적으로 나타냈다 라는 것은, 개발자가 코드를 작성할때 어떠한 변수에 값이 없다라는 것 을 표현하기 위해 사용했다고 볼 수 있다.
또한 undefined와는 다르게 사용되며 둘의 차이점은 undefined는 값이 정의되지 않은 값을 의미하고, null은 개발자가 의도적으로 값이 없다는 것을 표현한 것이다.
6 객체(Object) 와 배열(Array)
6-1. 객체(Object)
자바스크립트에서는 객체가 매우 중요한 역할을 한다.
객체(Object)는 속성과 메소드를 가지는 컨테이너로서 중괄호{} 를 사용하여 객체를 생성한다.
let person = {
name: 'Alice',
age: 20 ,
isMarried: true
};
console.log(person); // { name: 'Alice', age: 20, isMarried: true }
console.log(person.name); // Alice
console.log(typeof person); // object
console.log(person.age); // 20
console.log(typeof age); // undefined
console.log(person.isMarried); // true
console.log(typeof isMarried); // undefined
객체{} 안에는 키(key)와 밸류(value)를 넣어주어야 한다.
key 에는 따옴표 없이 문자형태로 입력, key 와 value를 이어주는 콜론: 을 사용하여 value를 넣어준다.
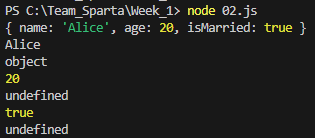
6-2. 배열(Array)
배열(Array)은 여러 개의 데이터를 순서대로 저장하는 데이터 타입이다.
대괄호[] 를 사용하여 배열을 생성하고, 인덱스(Index) 값을 가지고 있으며 대부분의 인덱스는 0부터 시작한다.
let number = [1, 2, 3, 4, 5];
console.log(number[0]); // 1
console.log(typeof number[0]); // number
console.log(number[1]); // 2
console.log(typeof number[1]); // number
console.log(number[2]); // 3
console.log(typeof number[2]); // number
console.log(number[3]); // 4
console.log(typeof number[3]); // number
console.log(number[4]); // 5
console.log(typeof number[4]); // number
console.log(number[5]); // undefined
console.log(typeof number[5]); // undefined

'프로그래밍' 카테고리의 다른 글
JavaScript 연산자 / 함수 (0) | 2024.04.24 |
---|---|
JavaScript 데이터타입 형변환 (0) | 2024.04.24 |
Node.js 변수의 5가지 주요 개념 및 특징 (0) | 2024.04.23 |
Node.js 설치하기 및 VS Code 에서 확인 (노드.js 설치하기) (0) | 2024.04.23 |
GitHub 협업하기 ( Git Clone ) (1) | 2024.04.18 |