자바스크립트에서는 다양한 자료형을 다룰 수 있고 이 자료형들은 서로 형변환이 가능하다.
이번에는 자바스크립트의 형 변환에 대해 공부해보자.
아래는 자바 스크립트의 형 변환 형태다.
1. 암시적 형 변환
1-1. 문자열 변환
1-2. 숫자 변환
2. 명시적 형 변환
2-1. Boolean 변환
2-2. 문자열 변환
암시적 형 변환은
- 개발자가 일부러 의도 하지 않은 변환
- 더하기+ 연산자가 나왔을 때는 문자열이 우선시 되어 변환
- 빼기-, 곱하기*, 나누기 / 연산자가 나왔을때는 숫자가 우선시 되어 변환되며, 문자 * 문자 와 같은 경우에는 숫자로 변환
명시적 형 변환은
- 개발자가 일부러 의도 한 변환
1. 암시적 형 변환
1-1. 문자열 변환
let result1 = 1 + "2";
console.log(result1); // 12
console.log(typeof result1); //string
숫자 1과 문자열 2를 더하고 출력을 하자, 숫자를 문자열로 변환하여 12 라는 문자가 출력되었다.
let result2 = "1" + true;
console.log(result2); // 1true
console.log(typeof result2); //string
문자열 1과 불리언 true를 더하고 출력을 하자, 문자열로 변환하여 1true 라는 문자가 출력되었다.
let result3 = "1" + {};
console.log(result3); // 1[object Object]
console.log(typeof result3); //string
문자열 1과 객체 {}를 더하고 출력을 하자, 문자열로 변환하여 1[object Object] 라는 문자가 출력되었다.
let result4 = "1" + null;
console.log(result4); // 1null
console.log(typeof result4); //string
문자열 1과 null을 더하고 출력을 하자, 문자열로 변환하여 1null 라는 문자가 출력되었다.
let result5 = "1" + undefined;
console.log(result5); // 1undefined
console.log(typeof result5); //string
문자열 1과 undefined을 더하고 출력을 하자, 문자열로 변환하여 1undefined 라는 문자가 출력되었다.
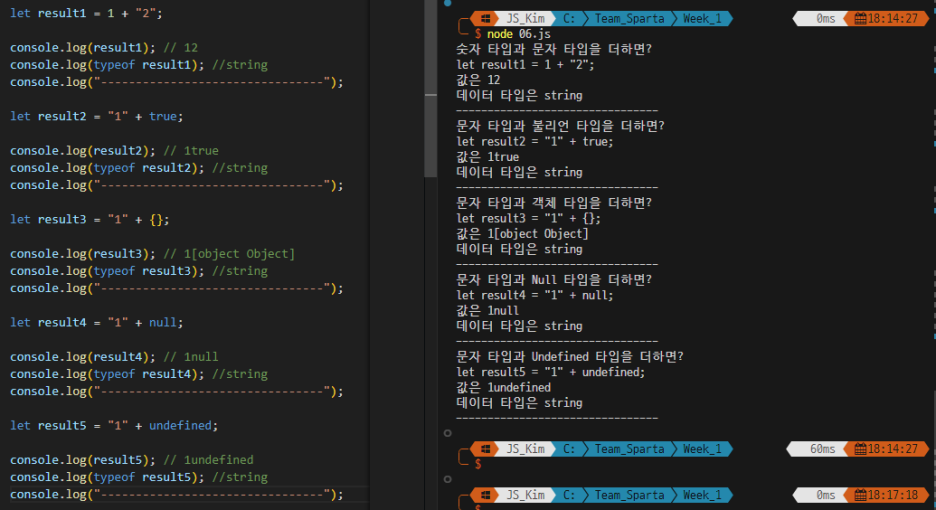
위 코드 처럼 문자열과 함께 다른 데이터 타입이 만나면 문자열로 변환이 되는데, 이를 암시적 변환 이라고 한다.
1-2. 숫자 변환
let result1 = 1 - "2";
console.log(result1); // -1
console.log(typeof result1); // number
숫자 1과 문자열 2를 빼고 출력을 하자, 문자를 숫자로 변환하여 -1 이라는 숫자가 출력되었다.
let result2 = "2" * "3";
console.log(result2); // 6
console.log(typeof result2); // number
문자열 2와 문자열 3을 곱하고 출력을 하자, 문자를 숫자로 변환하여 6 이라는 숫자가 출력되었다.
let result3 = "1" - {};
console.log(result3); // NaN
console.log(typeof result3); // number
문자열 1과 객체형을 빼고 출력을 하자, 객체를 숫자로 변환하여 NaN 이출력되었다.
let result4 = "1" * null;
console.log(result4); // 0
console.log(typeof result4); // number
문자열 1과 null을 곱하고 출력을 하자, null을 숫자로 변환하여 NaN 이출력되었다.
let result5 = "1" / undefined;
console.log(result5); // NaN
console.log(typeof result5); // number
문자열 1과 undefined을 나누고 출력을 하자, undefined을 숫자로 변환하여 NaN 이출력되었다.
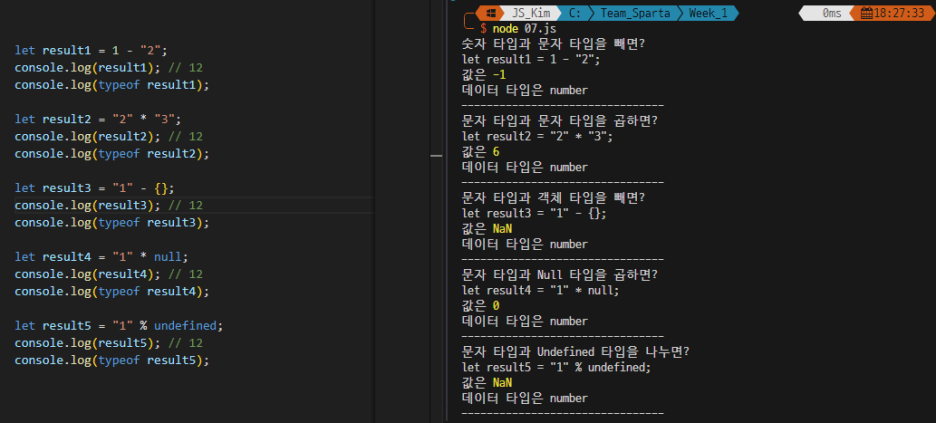
이처럼 빼기-, 곱하기*, 나누기% 연산자가 나왔을때 숫자가 우선시 되어 변환이 되고
문자 * 문자 와 같은 경우에도 숫자로 변환하여 계산이 된다.
2. 명시적 형 변환
명시적 형 변환은 기본적으로 어떠한 타입으로 변환을 하겠다 라고 적어주어야 한다.
명시적 형 변환은 아래와 같은 틀을 사용한다.
console.log(Boolean(0));
2-1. Boolean 변환
console.log(Boolean(0));
console.log(Boolean(""));
console.log(Boolean(null));
console.log(Boolean(undefined));
console.log(Boolean(NaN));
위와 같은 값들은 모두 Boolean 타입의 false가 출력된다.
console.log(Boolean("false"));
문자열의 내용이 false 라고 지정하여도, 문자열에 내용이 들어있기 때문에 true가 출력된다.
console.log(Boolean({}));
객체의 경우, 객체의 내부가 비어있더라도 true가 출력된다.
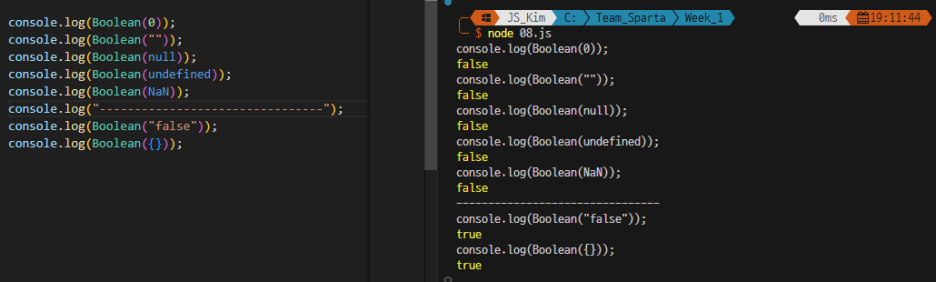
2-2. 문자열 변환
let result1 = String(123);
console.log(result1);
console.log(typeof result1);
숫자 123 을 넣고 출력을 하자, 문자열로 변환되어 123 이 출력되었다.
let result2 = String(true);
console.log(result2);
console.log(typeof result2);
true 를 넣고 출력을 하자, 문자열로 변환되어 true 가 출력되었다.
let result3 = String(false);
console.log(result3);
console.log(typeof result3);
false 를 넣고 출력을 하자, 문자열로 변환되어 false 가 출력되었다.
let result4 = String(null);
console.log(result4);
console.log(typeof result4);
null 을 넣고 출력을 하자, 문자열로 변환되어 null 이 출력되었다.
let result5 = String(undefined);
console.log(result5);
console.log(typeof result5);
undefined 을 넣고 출력을 하자, 문자열로 변환되어 undefined 이 출력되었다.

2-3. 숫자 변환
let result1 = Number("123");
console.log(result1);
console.log(typeof result1);
문자열 123 을 넣고 출력을 하자, 숫자로 변환되어 123 이 출력되었다.
let result2 = Number(true);
console.log(result2);
console.log(typeof result2);
true 를 넣고 출력을 하자, 숫자로 변환되어 1 이 출력되었다.
let result3 = Number(false);
console.log(result3);
console.log(typeof result3);
false 를 넣고 출력을 하자, 숫자로 변환되어 0 이 출력되었다.
let result4 = Number(null);
console.log(result4);
console.log(typeof result4);
null 을 넣고 출력을 하자, 숫자로 변환되어 0 이 출력되었다.
let result5 = Number(undefined);
console.log(result5);
console.log(typeof result5);
undefined 을 넣고 출력을 하자, 숫자로 변환되어 NaN 이 출력되었다.
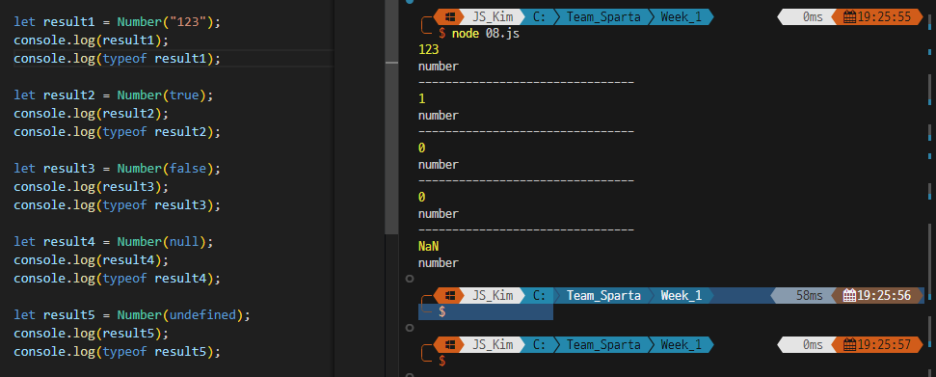
'프로그래밍' 카테고리의 다른 글
JavaScript 조건문 과 falsy한 값과 truthy한 값 (0) | 2024.04.25 |
---|---|
JavaScript 연산자 / 함수 (0) | 2024.04.24 |
변수의 데이터 타입 ( 숫자 / 문자열 / 불리언 / 객체 / 배열 / undefined / null ) (0) | 2024.04.23 |
Node.js 변수의 5가지 주요 개념 및 특징 (0) | 2024.04.23 |
Node.js 설치하기 및 VS Code 에서 확인 (노드.js 설치하기) (0) | 2024.04.23 |